Keep It Short: Taming Long Methods for Cleaner Code
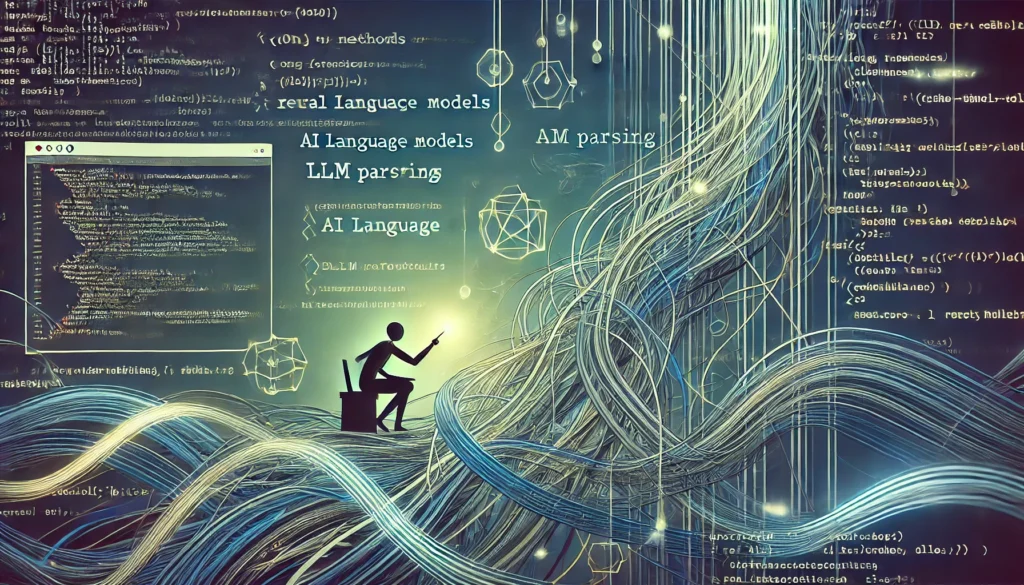
At Numberz.ai, we believe in building a strong relationship with our code, no matter how few lines it may be. Our guiding principles are clarity, testability, and relentless pursuit of perfection.
Most code smells are simple to spot and even easier to fix, but doing so requires unwavering dedication and a keen eye for detail. We embrace what we call “merciless refactoring,” a process that demands continuous attention and improvement.In this upcoming series of blogs, we’ll be delving into practices that enhance code readability, maintainability, and testability. We draw heavily on the wisdom shared in essential readings like Test Driven Development by Kent Beck, The Pragmatic Programmer by Andrew Hunt, and Refactoring by Martin Fowler. These books are indispensable for anyone serious about writing clean, effective code.
Lets Start
If you’ve ever found yourself scrolling endlessly through a method, struggling to understand its purpose, you’ve likely encountered the infamous Long Method code smell. This particular “bloater” is more than just an inconvenience—it’s a signal that your code might be doing too much in one place.
What is a Long Method? A method that tries to accomplish too much is often overly long and complex. It’s harder to read, maintain, and debug. Long methods can lead to duplicated code, increased error rates, and more technical debt over time.
Imagine you’re working on a RAG pipeline to parse a lengthy PDF, chunking its content, and storing it in a VectorDB for quick retrieval.
You then look up user queries in the chat, matching them against your VectorDB. Sounds straightforward, right? But what if your method responsible for all these tasks grows so large that it becomes a tangled mess of logic?
Welcome to the world of the Long Method code smell—where complexity lurks in the shadows, waiting to trip you up.
The Anatomy of a Long Method
A Long Method is like a Swiss army knife that’s trying to be everything at once. It’s a method that juggles too many responsibilities, making it harder to read, maintain, and test. In the context of RAG pipeline, this could be a method that handles everything from parsing and chunking the PDF to writing the data to the VectorDB and matching user queries.
The problem?
This method does way too much! It handles everything from validation to generating invoices and even logging—each of which could be a method on its own.
Why Long Methods Hurt Your LLM Pipeline
Long methods are more than just inconvenient—they’re a breeding ground for errors. They make your codebase more fragile, harder to debug, and nearly impossible to test in isolation.
This is especially problematic in an LLM pipeline, where the flow of data from one process to another needs to be seamless. A bug in one part of your Long Method could disrupt the entire pipeline, leading to inaccurate results or even system failures.
Consider this method:
public void processLLMTask(PDF pdf) {
parsePDF(pdf);
chunkContent(pdf);
writeToVectorDB(pdf);
lookupChatQueries(pdf);
matchVectors(pdf);
}
Here, we’re doing everything in one go—parsing the PDF, chunking its content, writing to the VectorDB, and then looking up and matching user queries. This method is not just long; it’s an epic saga with too many plot twists.
The Refactoring Mindset: Breaking Down the Monolith
To bring order to this chaos, we need to apply a refactoring strategy that Martin Fowler, Andrew Hunt, and others have championed for years: Extract Method. By breaking down our Long Method into smaller, focused methods, we isolate each responsibility, making the code easier to test, maintain, and extend.
Refactored Version:
public void processLLMTask(PDF pdf) {
ParsedContent content = parseAndChunkPDF(pdf);
writeToVectorDB(content);
matchChatQueries(content);
}
private ParsedContent parseAndChunkPDF(PDF pdf) {
ParsedContent content = parsePDF(pdf);
return chunkContent(content);
}
private void writeToVectorDB(ParsedContent content) {
// code to write content to VectorDB
}
private void matchChatQueries(ParsedContent content) {
// code to match user queries with VectorDB
}
By refactoring, we’ve transformed the method into a series of well-defined steps. Each step is now easier to understand and test, which is crucial in an LLM pipeline where precision and reliability are key.
The Pragmatic Programmer’s Take: Think of the Future
As The Pragmatic Programmer suggests, think about the long-term implications of your code. Today’s quick fix could be tomorrow’s nightmare. By breaking down your Long Method into manageable pieces, you’re not just improving your current project—you’re laying the groundwork for easier maintenance and scalability in the future.
In the world of LLMs, where processing pipelines can grow complex, keeping your methods small and focused isn’t just good practice—it’s essential. Refactor ruthlessly, and your future self (and your team) will thank you.
Conclusion
Refactoring isn’t just about cleaning up messy code—it’s about making your codebase resilient, readable, and ready for whatever comes next. When working with LLMs and complex pipelines, breaking down Long Methods is a small step that leads to significant improvements. So, take the time to refactor and let your code breathe—you’ll find that it becomes not just easier to manage, but also more powerful in its simplicity.
Future
We will be looking into methods which we should not refactor – for example something like Atomic Operations, highly cohesive methods etc. We will cover that in the next.
Leave a Reply