Code that resists change is destined to fail.
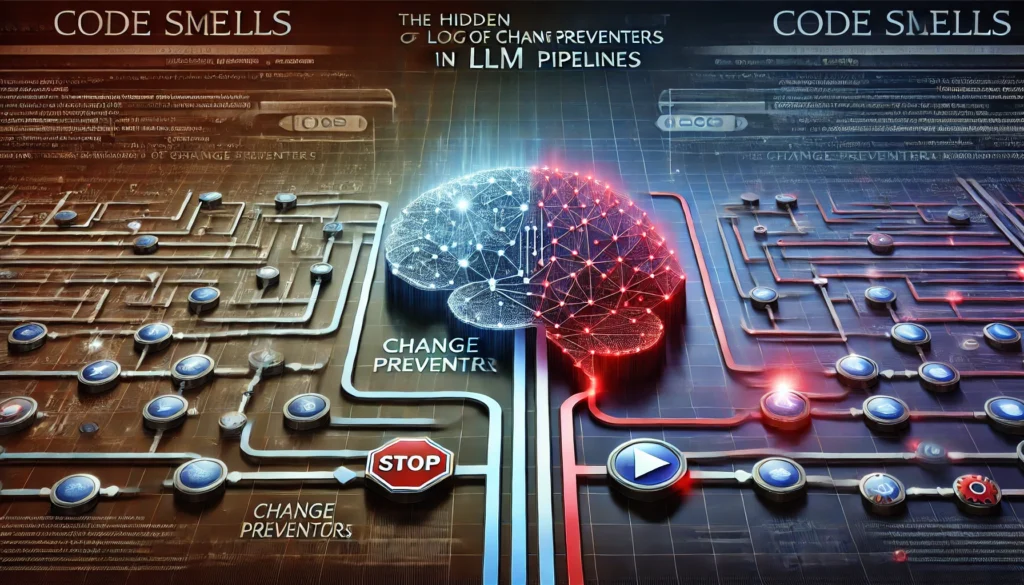
Numberz.ai, we believe in building a strong relationship with our code, no matter how few lines it may be. Our guiding principles are clarity, testability, and relentless pursuit of perfection.
Most code smells are simple to spot and even easier to fix, but doing so requires unwavering dedication and a keen eye for detail. We embrace what we call “merciless refactoring,” a process that demands continuous attention and improvement.
In this upcoming series of blogs, we’ll be delving into practices that enhance code readability, maintainability, and testability. We draw heavily on the wisdom shared in essential readings like Test Driven Development by Kent Beck, The Pragmatic Programmer by Andrew Hunt, and Refactoring by Martin Fowler. These books are indispensable for anyone serious about writing clean, effective code.
In the fast-evolving world of software development, particularly when working with complex systems like Large Language Models (LLMs), certain code smells can quietly infiltrate your codebase, gradually eroding its quality. One of the most insidious of these is the “Change Preventers” code smell—a subtle yet powerful force that can significantly hinder your ability to evolve and maintain your code over time.
What Are Change Preventers?
Change Preventers are parts of the code that make it difficult, if not dangerous, to modify or extend functionality. They often manifest as rigid and overly complex structures, where even minor changes can become risky and time-consuming. In the context of LLM pipelines, these smells can seriously impact your ability to keep up with changing requirements or improve your system’s performance.
Common examples of Change Preventers includes:
- God Objects: Classes or modules that take on too many responsibilities, often becoming monolithic and unmanageable.
- Tightly Coupled Code: Where components are so interdependent that making changes to one part necessitates widespread modifications elsewhere.
- Hardcoded Logic: Fixed values and deeply embedded logic that make the code difficult to adapt or reconfigure.
The cost of Change Preventers in LLM pipelines
Let’s bring this into the realm of LLM pipelines. Imagine you have a LLMPipelineManager class that handles everything—PDF parsing, chunking content, storing data in a VectorDB, and matching chat queries. Initially, this might seem efficient, but as your pipeline grows, this class becomes a tangled web of logic. Now, making a simple change to the chunking algorithm could inadvertently disrupt how data is stored in the VectorDB or how queries are matched. This is a classic God Object and a textbook example of a Change Preventer.
public class LLMPipelineManager {
public void handlePDFProcessing(PDF pdf) {
parsePDF(pdf);
chunkContent(pdf);
writeToVectorDB(pdf);
matchChatQueries(pdf);
}
private void parsePDF(PDF pdf) {
// PDF parsing logic
}
private void chunkContent(PDF pdf) {
// Content chunking logic
}
private void writeToVectorDB(PDF pdf) {
// Writing content to VectorDB
}
private void matchChatQueries(PDF pdf) {
// Matching user queries with VectorDB
}
}
In this scenario, the LLMPipelineManager has become a God Object, tightly coupling multiple responsibilities into a single class. The cost? Your ability to make changes becomes severely hampered, and the risk of introducing bugs skyrockets.
Why are Change Preventers a problem?
In today’s agile development environment, the ability to quickly adapt to changing requirements is crucial. Change Preventers slow down this process, increase technical debt, and ultimately impact the delivery of value to your end-users. In an LLM pipeline, where precision and adaptability are key, Change Preventers can be particularly detrimental, causing delays in feature updates, making it harder to optimize performance, and increasing the likelihood of errors.
How to combat Change Preventers
Refactor Ruthlessly: Regularly review and improve your codebase. Break down large, unwieldy classes like LLMPipelineManager into smaller, more focused components. Decouple tightly bound modules and eliminate hardcoded logic by introducing configuration files or dependency injection.
Refactored Example:
public void handlePDFProcessing(PDF pdf) {
ParsedContent content = parseAndChunkPDF(pdf);
storeContent(content);
processChatQueries(content);
}
private ParsedContent parseAndChunkPDF(PDF pdf) {
return pdfParser.parse(pdf).chunk();
}
private void storeContent(ParsedContent content) {
vectorDB.store(content);
}
private void processChatQueries(ParsedContent content) {
queryMatcher.match(content);
}
Write Comprehensive Tests: Ensure that your codebase has thorough test coverage. Tests give you the confidence to refactor code and introduce changes without the fear of breaking existing functionality. In an LLM pipeline, where changes can have far-reaching effects, this is especially important.
Embrace SOLID Principles: Design your classes and modules in a way that is scalable and adaptable to change. By adhering to principles like Single Responsibility and Dependency Inversion, you create a codebase that is more flexible and resilient to change.
Conclusion
Don’t let your codebase become a liability. Change Preventers might start as minor inconveniences, but over time, they can evolve into significant roadblocks that hinder your ability to innovate and respond to new challenges. By being vigilant, proactive, and willing to refactor when necessary, you can keep your LLM pipeline—or any system—flexible, maintainable, and ready for whatever the future holds.
Future
Using design patterns like Command, Chain of Responsibility, or Strategy helps mitigate the “Change Preventers” code smell by promoting better organization, reducing dependencies, and making the code more maintainable. Each of these patterns provides a structured approach to managing complex operations in an LLM pipeline, making your system more adaptable to change and easier to extend as new requirements arise. We will cover that in our future blogs.
Leave a Reply